Nitric Documentation
Build cloud backends that run anywhere, fast.
Nitric is a declarative cloud framework with common resources like APIs, websockets, databases, queues, topics, buckets, and more.
However, Nitric doesn't just deploy the resources, it helps you interact with them. It also makes them pluggable, so you can swap services or even whole clouds without changing your code.
It's what's missing between applications and infrastructure automation.
Oh, and it supports basically any language, like JavaScript, TypeScript, Python, Go, you name it.
import { api } from '@nitric/sdk'const main = api('main')main.get('/hello/:name', async ({ req, res }) => {const { name } = req.paramsctx.res.body = `Hello ${name}`})
If you're already familiar with Nitric, you might want to jump to the Installation, Guides or Resources sections. If you're new to Nitric, consider starting with the Why Nitric page, or the Quick Start guide if you want to jump straight into the action.
Otherwise, keep reading to learn more about the core elements of Nitric.
Services
Services are the heart of Nitric apps, they're the entrypoints to your code. They can serve as APIs, websockets, schedule handlers, subscribers and a lot more. You create services by telling Nitric where to look for your code and how to run it.
name: exampleservices:- match: services/*.tsstart: npm run dev:services $SERVICE_PATH
You might have one service that handles everything, or a service for each route. It's up to you. Every matched service becomes a container, allowing them run and scale independently.
Resources
Resources are the building blocks of your apps. They're the databases, queues, buckets, etc. that your services interact with. To request a resource, import the resource type and create one with a name.
import { bucket } from '@nitric/sdk'const profiles = bucket('profiles').allow('read', 'write', 'delete')
Nitric collects everything your services request. When you deploy, the deployment plugin you choose creates the resources and services, then links them all together.
Deployment Plugins (Providers)
Nitric is designed to be independent of any platform, so you can run your apps anywhere. You can deploy to AWS, Azure, GCP, your own Kubernetes cluster or a single server. You could even deploy to multiple clouds at once.
Are the differences between a bucket on AWS and a bucket on Azure important to most apps? We don't think so. So why should the code be different?
Nitric abstracts away API layer differences, so you can focus on your app. The part that makes that possible is a plugin, we call a Provider.
provider: nitric/aws@1.1.1region: us-east-1
We have several providers built-in with IaC from Pulumi or Terraform, but you can build your own with any tools you prefer and deploy anywhere.
Projects
Projects built with Nitric don't have many restrictions. You can use most languages, libraries, tools, clouds, services, mostly anything you like. But, you need to have a nitric.yaml
file in the root of your project.
name: exampleservices:- match: services/*.tsstart: npm run dev:services $SERVICE_PATH
Nitric uses this to find your services, then it turns each service into a container, runs them in deployment mode to match the resources you requested and gives the result to the Provider - which either generates IaC (like Terraform) or automatically deploys your app.
So, a project structure might look something like this:
You can read more about how Nitric Projects work in the Foundations section.
CLI
Nitric has a CLI to help you create, manage, run and deploy your projects. We recommend installing it with one of the options below:
brew install nitrictech/tap/nitric
Nitric has a few dependencies, like Docker, which you can read about in the Installation section.
New
You can create a new project from a template with the new
command.
nitric new
Start
You can run your project locally with the start
command.
nitric start
Start also emulates the resources you requested, so you can test your app locally. It also provides a Dashboard UI to interact with the resources.
You can read about Nitric's Local Development features in the Foundations section.
Deploy
You can deploy your project with the up
command, once you have a stack file (deployment target).
# Create a new stack (deployment target)nitric stack new# Deploy to the stack, using the provider in the stack filenitric up
Some providers deploy directly, others generate IaC files for existing tools
(like HCL for Terraform). In the cases where IaC is generated you can use
your preferred IaC tool to deploy (e.g. terraform apply
).
Currently, all Pulumi providers deploy directly, while Terraform providers generate IaC.
You can read more about Nitric Providers in the Foundations section.
Tear Down
You can tear down your project with the down
command.
nitric down
If you used a provider that generates IaC, use the IaC tool to tear down (e.g.
terraform destroy
).
We recommend reading more about Nitric Deployments in the Foundations section.
Local development
Sometimes you'll want to run your app locally. We don't mean "binds to a cloud environment and syncs your code, but doesn't work without Wifi" local, we mean "runs on your machine, on a desert island" local.
nitric start
hosts entrypoints like APIs, websockets, and schedules, as well as resources like databases, topics, queues, key/value stores and buckets.
It also provides a Dashboard to interact with your resources, so you can trigger schedules without waiting, or topics without a publisher, or upload test files to a bucket. It even produces a live architecture diagram of your services and resources as your code updates.
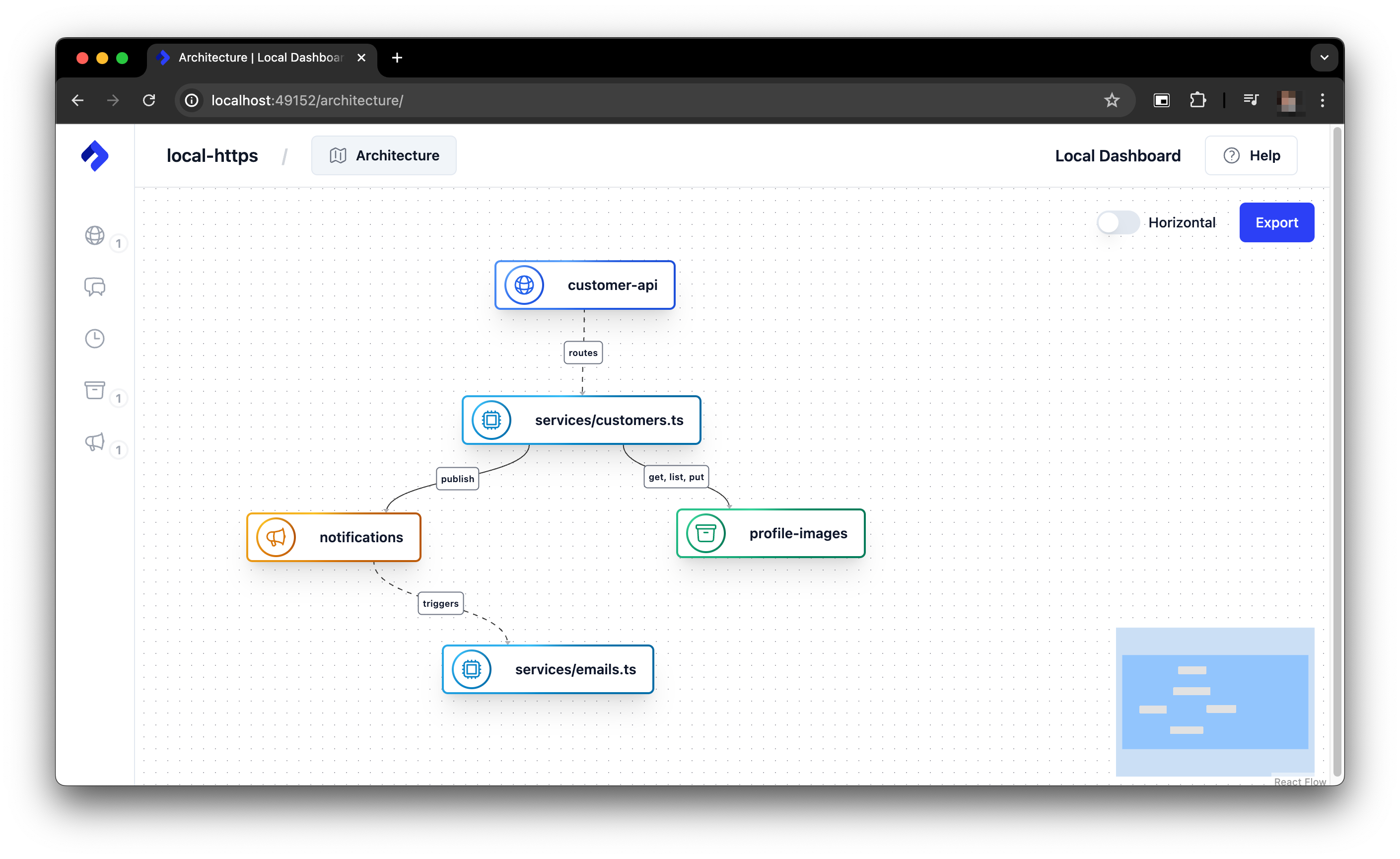
Extension & Escape Hatches
The services of cloud providers are vast, we can't cover everything and we shouldn't. Many services are unique enough that abstraction would be a disservice. Nitric aims to make common "table stakes" services easy to use, but we never want to limit you.
Here are some ways you can extend or escape Nitric.
Runtime
If you need to access a service in a way Nitric doesn't support, you can always use the provider's SDK directly. Code like this will always work:
// Import the AWS SDKimport { S3Client } from '@aws-sdk/client-s3'// Use it like you normally wouldconst s3 = new S3Client({ region: 'us-east-1' })const { Contents } = await s3.listObjectsV2({ Bucket: 'my-bucket' })
Overriding
If you need to change how Nitric deploys a resources or how it interacts with a service at runtime, you can extend or modify a provider.
For example, here's a project that swaps SNS for EventBridge on AWS.
Full Customization
If you need to deploy to a new platform or new set of services that Nitric doesn't support, you can build your own provider. This is a bit more advanced, but it's the ultimate escape hatch.
The included providers are written in Go and built using Terraform or Pulumi, but you can use any language or tool you like.
Additional Resources
If you need to use a service/resource that Nitric doesn't support, you do that like you always would. Nitric doesn't get in the way of you using the cloud, it just makes it easier.
What's next
- Start building with the quick start guide.
- Checkout out the foundations section for a more detailed introduction to Nitric.
- Dive into the guides section for more in-depth tutorials.