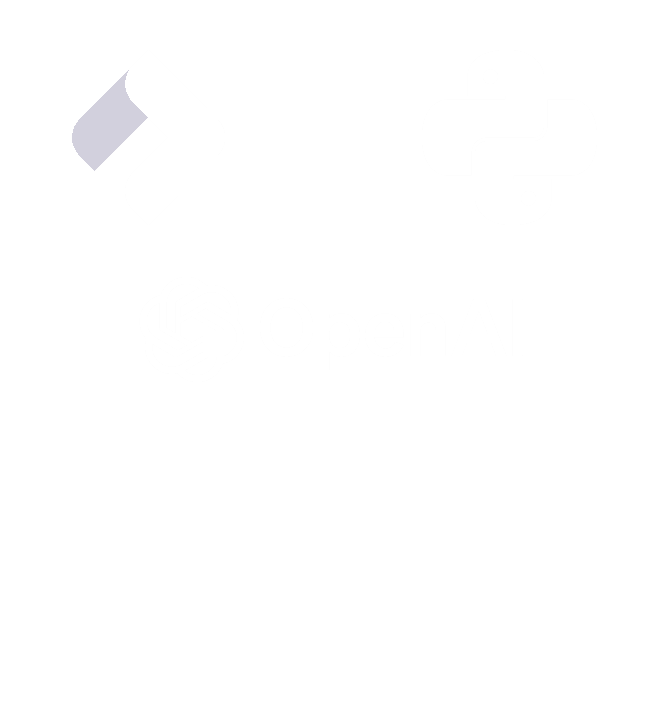
Build a Serverless AI-Powered Python API
Build a Serverless AI-Powered Python API
An open source universal backend framework, with concise infrastructure and pluggable deployment automation.
import { api, bucket } from "@nitric/sdk";const main = api("main");const notes = bucket("notes").allow("read", "write");main.get("/notes/:title", async ({req, res}) => {const { title } = req.params;res.body = await notes.file(title).read();});main.post("/notes/:title", async ({req, res}) => {const { title } = req.params;await notes.file(title).write(req.text());});
Nitric is a multi-language backend framework that lets you declare infrastructure requirements in code for common cloud resources and provides a convenient interface to interact with them.
Using Terraform, Pulumi, or any tool you prefer, Nitric plugins automate cloud resource provisioning, security and linking. Nitric provides plugins for AWS, Azure and Google Cloud out of the box, then extend or build your own when things get custom.
You can easily create APIs using Nitric. With a familiar syntax, it's simple to define your routes, middlewares, and handlers all from the comfort of your application code.
import { api } from "@nitric/sdk"const main = api("main")main.get("/hello/:name", (ctx) async {const { name } = ctx.req.paramsctx.res.body = `Hello ${name}`return ctx;})
Writing authentication and authorisation for your API is as simple as adding security definitions to your API resource. Integrates with any OIDC-compatible providers such as Auth0, FusionAuth, and AWS Cognito.
import { api, oidcRule } from '@nitric/sdk'const defaultSecurityRule = oidcRule({name: 'default',audiences: ['https://test-security-definition/'],issuer: 'https://dev-abc123.us.auth0.com',})const secureApi = api('main', {security: [defaultSecurityRule('user.read')],})
APIs automatically deploy to API Gateway services like AWS API Gateway, Google Cloud API Gateway, or Azure API Management.
Route handlers are automatically built as containers and deployed to serverless container runtimes like AWS Lambda, Google CloudRun, or Azure Container Apps
Access high-performance data and AI services like AWS Batch, Google Cloud Batch and Azure Batch using the Nitric Batch resources.
Completely customize the resources required for your long running jobs and access the latest GPUs that the clouds have to offer.
import { job } from "@nitric/sdk"const genImages = job("generate-image");genImages.handler(async (data) => {// do something amazing},{ cpus: 4, memory: 16384, gpus: 1 },);
Send tasks to batch services from any other service.
await genImages.submit({});
Deploy Postgres SQL databases with 1 line of code to managed database services like AWS RDS, Google Cloud SQL or Azure Database for PostgreSQL with Nitric.
import { database } from "@nitric/sdk";import { client } from "your-database-client";import { users } from "../schema";const db = database("db");const getDbClient = async () => {const connectionString = db.connectionString();return client(connectionString);};const addUser = async (user) => {const db = await getClient();await db.insert(users, user);};
Migrations act as version control for your database, enabling progressive schema updates without the worry.
const db = database("db", {migrations: "file://migrations",});
Read, write, and delete large files to serverless object stores like Amazon S3, Google Cloud Storage, or Azure Blob Storage with Nitric's Bucket API.
import { bucket } from '@nitric/sdk'const profiles = bucket('profile-images').allow('write')await profiles.file('profiles/user-343.jpg').write(imageData)
Define reactive eventing for your storage workflows using Bucket Notifications.
import { bucket } from '@nitric/sdk'const profiles = bucket('profile-images')profiles.on('delete', '*', (ctx) => {console.log(`${ctx.req.key} image was deleted`)})
Implement pub/sub or queue-based messaging for real-time event handling, fan-out, task-driven workloads and more.
Nitric supports many popular Queue and Topic services, such as Amazon SQS, Amazon SNS, Google Pub/Sub, Azure Event Grid and Azure Storage Queues.
For pub/sub, define Topics, Publishers and Subscribers, then publish messages to a topic and have them reactively handled by subscribers.
import { topic, api } from "@nitric/sdk";const events = topic("events").allow("publish");// Publish messages from any service, such as APIs.api('public').post('/users', async ({req, res}) => {const userData = req.json()await events.publish({type: 'user.created',details: userData,})}
Define subscribers to handle events asynchronously.
import { topic } from "@nitric/sdk";const events = topic("events");events.subscribe(async (ctx) => {// Extract data for processingconst { details } = ctx.req.json()logEvent(details)})
If you want to offload processing of messages from your main API calls you can use a Queue and write a service to handle events separately.
import { queue } from '@nitric/sdk'const transactionQueue = queue('transactions').allow('enqueue')await transactionQueue.enqueue({message: 'hello world',})
Build apps with presence, realtime and multiplayer collaboration using WebSockets. Backed by cloud services like Amazon API Gateway.
Nitric's websockets allow you to send and listen for messages between services, and connections can be stored using a Nitric Key Value Store resource.
import { websocket, kv } from '@nitric/sdk'const conns = kv('connections').allow('get')const socket = websocket('presence')// Broadcast updatessocket.on('message', async (ctx) => {for await (const connectionId of conns.keys()) {await socket.send(connectionId, ctx.req.text())}})
Build and deploy scheduled tasks and jobs to run at specific times or intervals, written in plain language.
import { schedule } from '@nitric/sdk'const process = schedule('process-transactions');process.every('15 minutes', async () => {processTransaction()})
Or you can get custom and use Cron scheduling, up to you.
process.cron('*/15 * * * *', async () => {processTransaction()})
Scheduled services automatically build and deploy to container runtimes like AWS Lambda, Google CloudRun, or Azure Container Apps
Easily store, update, and retrieve sensitive information like database credentials and API keys, while securely managing access to your secrets.
import { secret } from '@nitric/sdk'// Create a new secretconst apiKey = secret('api-key').allow('put')// Store a new valueconst latestVersion = await apiKey.put('a new secret value')
You can securely access the latest version of a secret and retrieve its value.
import { secret } from '@nitric/sdk'const apiKey = secret('api-key').allow('access')// Access the details of the latest version of a secretconst latest = await apiKey.latest().access()// Retrieve the value of the secret as a stringconst value = latest.asString()
$ nitric start
"As the founder and publisher of TNS, I see development and infrastructure trends come and go, while core technologies and challenges remain. Development and operations teams have been struggling for years to bridge the gap between them, and I'm convinced that Nitric has what it takes. They are positioned to be a leader in what we see as what will be critical to transform cloud development."
"The @nitric_io folks are always a joy to talk to... they pour the same enthusiasm and empathy into their product as the developers who use it."
"@nitric_io is indeed an amazing service, our team spent a lot of time deploying and debugging multiple lambda's, especially on testing AWS with Pulumi, Nitric's out of the box and 0 configuration was a great fit for us."
"Building my multi-tenant solution with @nitric_io freed me up to focus on the application code instead of how to deploy infrastructure for scale and configure each of the cloud services to work correctly. Nitric saved me weeks of effort on infrastructure, and their hot-reloading let me rapidly iterate my code during local development."
"@nitric_io has completely transformed our developer productivity so that we can deliver business value quickly. Taking our lengthy deployment process to a fast, fully-automated pipeline has a real impact on the cost to our business and gives us a competitive edge in our speed to market. We're well positioned now to quickly release new features and go after big business opportunities, all while cutting our AWS hosting costs."
"@nitric_io's local development experience has been a game-changer for our team. Previously, working with SAM locally was cumbersome and inefficient. The time-consuming and costly process of debugging in the cloud had us constantly searching for a better solution. Nitric allowed us to build, test, and debug our app offline with ease, enabling us to deploy to the cloud only when we were confident in its readiness. This streamlined approach has significantly enhanced our productivity."
"It was a pleasure contributing to @nitric_io 🙌"
"Working with the @nitric_io framework is fun and productive; API creation is seamless, and the overall developer experience is enjoyable. Our team has achieved much easier infrastructure management with Nitric, and our build and deploy times are magnitudes faster. As a result, we can create and release features in half the time, and we'll save even more time on future features that would have required a lot of DevOps work without Nitric."
"@nitric_io is a game changer. I can use it to deliver working API endpoints on day 1, and that's awesome for building trust with a client. Plus, because Nitric is cloud-agnostic, I can de-risk my choices and stay focused on moving fast. This is the best developer experience I've ever seen."
"Awesome cloud/app building experience, a game changer."
"As I've worked on passion projects and experimented with new technology, @nitric_io has been a great framework to help me build and deploy apps without having to be an expert in any particular cloud provider. By leaning on Nitric's abstraction, I can stay focused on domain logic and get a working project to market much faster. Nitric also makes it so I can defer my cloud vendor choice and have a low-cost, low-risk method for switching vendors at any point. For teams, Nitric makes it easy to fit into infrastructure guardrails established by previous projects and eliminates a lot of rework and glue code."
"A big shoutout to the @nitric_io team (@JyeCusch, @stevedemchuk, @timjholm, @raksiv_, and @davemooreuws) for the amazing tool and for the phenomenal support they provided for launching #Python v1 and for addressing the GitHub Issues 💯🙌✅"
ship faster with next generation infrastructure automation using nitric.